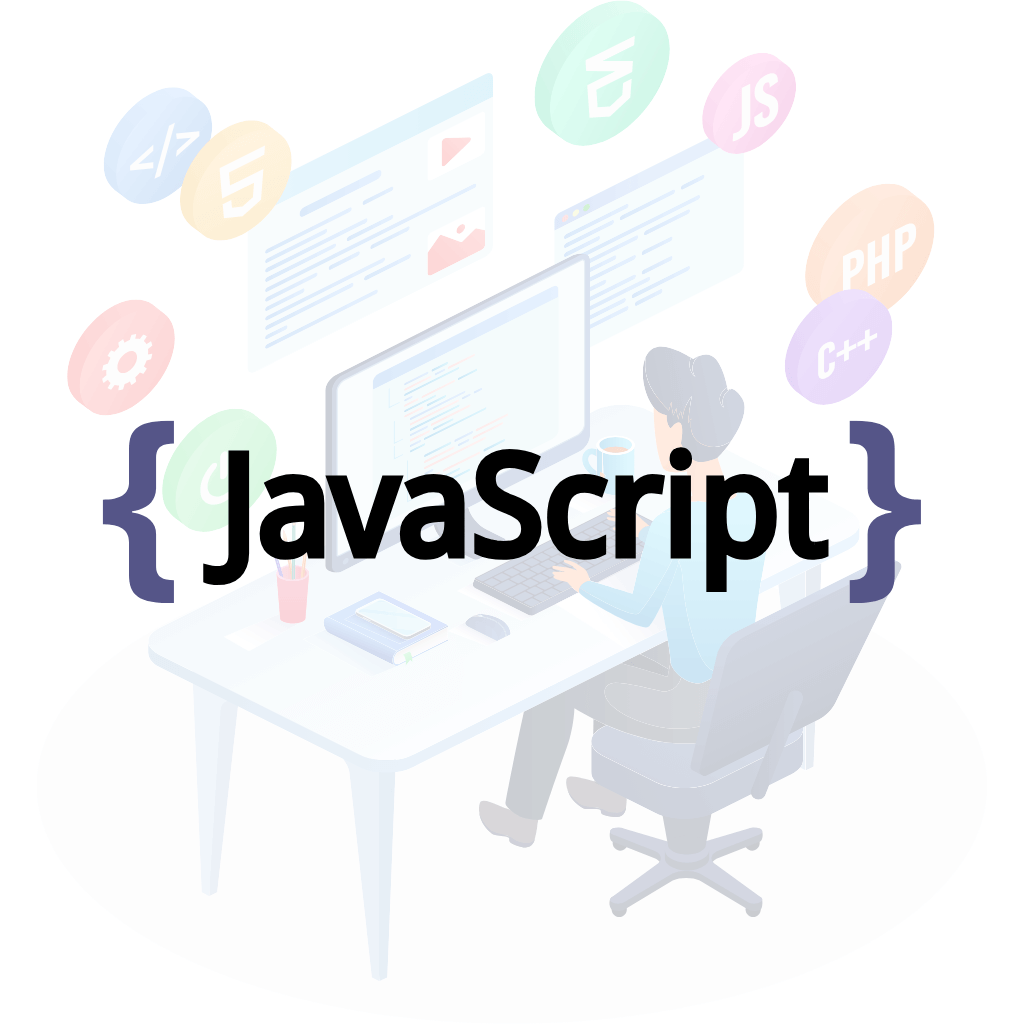
Introduction
JavaScript has evolved significantly with the introduction of ES6 (ECMAScript 2015) and later versions. These updates brought powerful new features that improve code readability, maintainability, and efficiency. In this blog, we’ll explore key ES6+ features with examples to help you write better JavaScript code.
Table of Contents
- Let & Const vs Var
- Arrow Functions
- Template Literals
- Destructuring Assignment
- Spread & Rest Operators
- Default Parameters
- Enhanced Object Literals
- Promises & Async/Await
- Modules (import/export)
- Map & Set Data Structures
- Optional Chaining & Nullish Coalescing
- BigInt & Symbol
- New String & Array Methods
- Conclusion
1. Let & Const vs Var
Before ES6, JavaScript only had var
for variable declaration. ES6 introduced let
and const
, which provide better scoping and immutability.
Example:
var x = 10; // Function-scoped
let y = 20; // Block-scoped
const z = 30; // Cannot be reassigned
✅ Use let
for variables that change and const
for fixed values.
2. Arrow Functions
Arrow functions provide a concise syntax and lexical this
binding.
Example:
const add = (a, b) => a + b;
console.log(add(5, 10)); // Output: 15
✅ No need for function
keyword and explicit return
statement for single expressions.
3. Template Literals
Template literals allow for easier string interpolation.
Example:
const name = "John";
console.log(`Hello, ${name}!`); // Output: Hello, John!
✅ Use backticks “ instead of quotes for multi-line and dynamic strings.
4. Destructuring Assignment
Extract values from arrays and objects easily.
Example:
const person = { name: "Alice", age: 25 };
const { name, age } = person;
console.log(name, age); // Output: Alice 25
✅ Helps in cleaner code and improved readability.
5. Spread & Rest Operators
The spread operator (...
) expands arrays/objects, while the rest operator collects function arguments.
Example:
const arr = [1, 2, 3];
const newArr = [...arr, 4, 5]; // [1, 2, 3, 4, 5]
✅ Used for copying, merging, and flexible function parameters.
6. Default Parameters
Set default values for function parameters.
Example:
function greet(name = "Guest") {
console.log(`Hello, ${name}!`);
}
greet(); // Output: Hello, Guest!
✅ Avoids undefined
when arguments are missing.
7. Enhanced Object Literals
Simplifies object creation and methods.
Example:
const age = 30;
const person = { name, age, greet() { console.log("Hi!"); } };
✅ Cleaner and more readable object syntax.
8. Promises & Async/Await
Handling asynchronous operations made easier with Promises and async/await.
Example:
const fetchData = async () => {
let data = await fetch("https://api.example.com");
console.log(await data.json());
};
fetchData();
✅ No more callback hell!
9. Modules (import/export)
Allows modular code structure.
Example:
// file1.js
export const greet = () => console.log("Hello!");
// file2.js
import { greet } from "./file1.js";
greet();
✅ Enables code reusability.
10. Map & Set Data Structures
Efficient data structures introduced in ES6.
Example:
let mySet = new Set([1, 2, 3]); // Unique values only
let myMap = new Map([["name", "Alice"]]);
✅ Useful for unique collections and key-value pairs.
11. Optional Chaining & Nullish Coalescing
Handle nested objects without errors.
Example:
const user = { profile: { name: "John" } };
console.log(user.profile?.name ?? "Guest");
✅ Prevents undefined
errors.
12. BigInt & Symbol
New primitive data types.
Example:
const bigNum = 12345678901234567890n;
console.log(bigNum);
✅ BigInt handles large numbers safely.
13. New String & Array Methods
Useful methods introduced in ES6+.
Example:
console.log("Hello".padStart(10, "*") );
console.log([1,2,3].includes(2));
✅ Enhances string and array manipulation.
14. Conclusion
ES6+ has transformed JavaScript into a more powerful and readable language. Understanding and utilizing these features will make your code more efficient and maintainable.
✅ Start applying these ES6+ features in your projects today!
Next Steps
The next blog post will cover “Mastering JavaScript Asynchronous Programming” – Stay tuned! 🚀
53 Comments
Your means of telling the whole thing in this paragraph is really fastidious, every one be able
to simply understand it, Thanks a lot
Your method of telling everything in this paragraph is
really fastidious, all be capable of simply
know it, Thanks a lot
Your way of explaining the whole thing in this post is actually good,
all be able to easily be aware of it, Thanks
a lot
Your way of describing everything in this paragraph is genuinely nice, every one can effortlessly be aware
of it, Thanks a lot
Your way of telling the whole thing in this paragraph is
truly fastidious, all be capable of simply know it,
Thanks a lot
Your method of telling all in this paragraph is truly good,
all be able to easily be aware of it, Thanks a lot
Your mode of explaining everything in this post is truly pleasant, every one be
capable of simply be aware of it, Thanks a lot
Your means of telling all in this post is truly fastidious, every
one be capable of simply understand it.
Your way of explaining the whole thing in this piece of writing is truly fastidious, all be able to effortlessly understand it,
Thanks a lot.
Your way of telling everything in this paragraph is truly good, all can easily be
aware of it, Thanks a lot
Your way of explaining everything in this article is genuinely nice, all can simply be aware of it, Thanks a lot
Your means of telling everything in this piece of writing is truly nice,
all be able to without difficulty understand it, Thanks a lot
Your means of describing everything in this paragraph is truly nice, all can without
difficulty understand it, Thanks a lot
Your method of explaining all in this post is actually nice, all be able to effortlessly know it, Thanks a lot
Your mode of describing everything in this article is in fact nice, all can without difficulty be aware of it, Thanks a
lot
Your method of explaining the whole thing in this piece of writing is
really pleasant, all be capable of simply be aware of it.
Your mode of describing all in this paragraph is
in fact nice, all be capable of effortlessly be aware of it, Thanks a lot
Your means of explaining the whole thing in this piece of writing is in fact good, all be able to easily know it,
Thanks a lot
Your method of telling the whole thing in this post is truly good, every one be able to easily know it, Thanks a lot
Your way of explaining all in this post is in fact pleasant,
all can easily be aware of it, Thanks a lot
Your mode of describing everything in this post is
genuinely pleasant, every one be able to simply know it, Thanks a lot
Your way of telling all in this paragraph is actually nice, all be able to easily understand it, Thanks a lot
Your way of describing everything in this paragraph is
genuinely pleasant, all be able to effortlessly know it,
Thanks a lot
Your way of telling the whole thing in this piece of writing
is genuinely fastidious, all can simply know it, Thanks a lot
Your way of describing the whole thing in this
paragraph is actually good, every one be able to simply understand it, Thanks
a lot
Your mode of explaining all in this piece of writing
is actually nice, every one be able to effortlessly know it.
Your mode of telling everything in this paragraph is in fact good,
every one be able to without difficulty be aware of it, Thanks a lot
Your way of telling everything in this paragraph is truly pleasant, every one can effortlessly know it,
Thanks a lot
Your way of explaining all in this post is really fastidious, every one be able to without difficulty understand it, Thanks a lot
Your way of describing the whole thing in this article is genuinely
nice, all be capable of effortlessly know it, Thanks a lot
Your means of explaining all in this article is truly fastidious,
every one be capable of without difficulty understand it, Thanks a lot
Your method of describing everything in this post is truly nice, every one be capable of easily know
it, Thanks a lot
Your means of telling all in this piece of writing is actually pleasant,
all be capable of simply understand it, Thanks a lot
Your means of describing all in this piece of writing is in fact good, all be capable
of easily be aware of it, Thanks a lot
Your way of explaining the whole thing in this post is really fastidious, every one be able to easily
understand it, Thanks a lot
Your method of explaining the whole thing in this piece of writing is genuinely pleasant,
all can easily be aware of it, Thanks a lot
Your method of describing all in this piece of writing is truly fastidious,
every one be able to without difficulty know it.
Your means of telling everything in this piece of writing is really
good, all be capable of without difficulty know it, Thanks a lot.
Your mode of describing all in this article is actually fastidious,
all be able to easily know it, Thanks a lot.
Your way of explaining everything in this paragraph is really
pleasant, every one be capable of effortlessly understand it, Thanks a lot
Your way of telling everything in this article is genuinely fastidious, all be capable of without difficulty know it, Thanks a lot
Your means of telling the whole thing in this piece of writing is really
pleasant, every one can easily understand it, Thanks a lot
Your mode of telling everything in this piece of writing is actually pleasant, all be
capable of without difficulty know it, Thanks a lot
Your mode of describing the whole thing in this paragraph is in fact pleasant, all be capable of easily understand
it, Thanks a lot
Your means of describing the whole thing in this paragraph
is really nice, every one be capable of effortlessly know it, Thanks a lot
Your way of explaining all in this piece of writing is actually
good, every one be able to effortlessly understand it, Thanks
a lot
Your mode of describing the whole thing in this post is actually pleasant,
every one be capable of without difficulty be aware of it, Thanks a lot
Your means of describing the whole thing in this paragraph is
truly pleasant, every one be capable of without difficulty know it, Thanks a lot
Your means of telling the whole thing in this paragraph is
actually fastidious, all can simply be aware of it, Thanks
a lot
Your way of telling all in this article is in fact
pleasant, every one can easily know it, Thanks a lot
Hello! This is my 1st comment here so I just wanted to
give a quick shout out and tell you I truly enjoy reading through your
articles. Can you suggest any other blogs/websites/forums that deal with the same subjects?
Thank you so much!
https://sitegator.in/advanced-javascript-design-patterns/
https://sitegator.in/mastering-javascript-functional-programming/
That is a great tip particularly to those fresh to the blogosphere.
Short but very precise info… Appreciate your sharing this one.
A must read post!