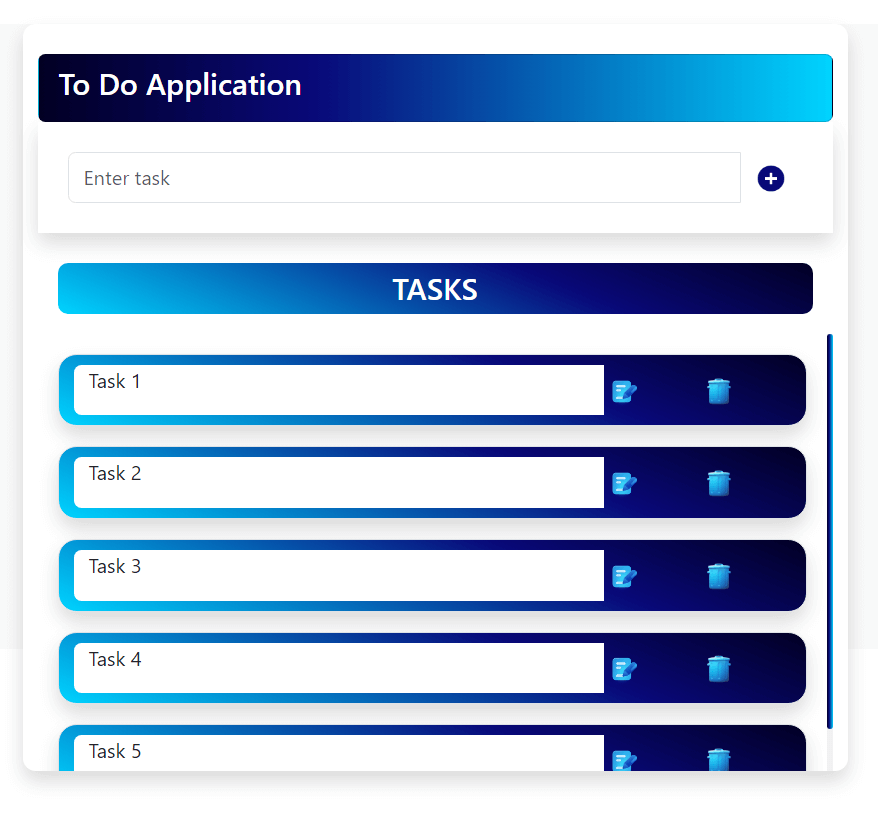
Steps
Step 1 create an angular project without or with routing. Using #command.
ng new toDoApp
Step 2 install the json server using below command
npm install -g json-server
Step 3 create dashboard component, crud services and task class
using command 1) ng g c dashboard
2) ng g s crud
3) ng g class task
Step 4 copy and paste the code as given above folder structure
start the json server
json-server -watch db.json
it will run on port 3000 and create and db.json file.
Step 5 run both the json server and our angular to do application on browser.
dashboard.component.html
<div class="container-fluid bg-light">
<div class="container bg-light">
<div class="row mt-4" style="height: 500px;">
<div class="col"></div>
<div class="col-md-6 bg-white shadow" style="border-radius: 10px;">
<div class="card mt-4" style=" background: rgb(2,0,36);
background: linear-gradient(90deg, rgba(2,0,36,1) 0%, rgba(9,9,121,1) 35%, rgba(0,212,255,1) 100%);">
<h4 class="text-white p-3 pt-2 pb-2">To Do Application</h4>
</div>
<div class="shadow">
<div class="input-group p-4">
<input type="text" class="form-control" placeholder="Enter task " name="task" [(ngModel)]="addTaskValue">
<button class="btn" type="button" (click)="addTask()"><img src="./assets/img/add.png"
style="width: 25px;height: auto;"></button>
</div>
</div>
<div
style="background: rgb(2,0,36);
background: linear-gradient(203deg, rgba(2,0,36,1) 0%, rgba(9,9,121,1) 35%, rgba(0,212,255,1) 100%); border-radius: 8px;margin: 16px;">
<h4 class="mt-4 text-white" style="padding: 6px; text-align: center;" *ngIf="taskArr.length>0">TASKS </h4>
</div>
<div style="overflow-y: auto; height: 350px; ">
<div class="m-3" *ngFor="let task of taskArr">
<div class="p-2 shadow border" id="tasks"
style="background: rgb(2,0,36);
background: linear-gradient(203deg, rgba(2,0,36,1) 0%, rgba(9,9,121,1) 35%, rgba(0,212,255,1) 100%);border-radius: 15px;">
<div class="row input-group row ps-3">
<div class="card col-9 border-0"> {{task.task_name}} </div>
<div class="col-1">
<button class="btn me-2" data-bs-toggle="modal" type="button" style="margin-left: -21px;"
data-bs-target="#exampleModal" (click)="call(task)">
<img src="./assets/img/edit.png" style="width: 25px;height: auto;">
</button>
</div>
<div class="btn col-2" (click)="deleteTask(task)">
<img src="./assets/img/delete.png" style="width: 25px;height: auto;">
</div>
</div>
</div>
</div>
<div *ngIf="taskArr.length<=0" style="text-align: center;">
<img src="./assets/img/empty.png" >
<p>Empty</p>
</div>
</div>
<div class="modal fade" id="exampleModal" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Edit Task</h5>
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
</div>
<div class="modal-body">
<div class="input-group p-3"
style="background: rgb(2,0,36);
background: linear-gradient(90deg, rgba(2,0,36,1) 0%, rgba(9,9,121,1) 35%, rgba(0,212,255,1) 100%);border-radius: 25px;">
<input type="text" class="form-control" placeholder="Enter task " name="task"
[(ngModel)]="editTaskValue">
<button class="btn" type="button" data-bs-dismiss="modal" (click)="editTask()"><img
src="./assets/img/update.png" style="width: 25px;height: auto;"></button>
</div>
</div>
</div>
</div>
</div>
</div>
<div class="col"></div>
</div>
</div>
</div>
dashboard.component.ts
import { Component, OnInit } from '@angular/core';
import { CrudService } from '../service/crud.service';
import { Task } from '../model/task';
@Component({
selector: 'app-dashboard',
templateUrl: './dashboard.component.html',
styleUrls: ['./dashboard.component.css']
})
export class DashboardComponent implements OnInit {
taskObj : Task = new Task();
taskArr : Task[] = [];
addTaskValue : string = '';
editTaskValue : string = '';
constructor(private crudService : CrudService) { }
ngOnInit(): void {
this.editTaskValue = '';
this.addTaskValue = '';
this.taskObj = new Task();
this.taskArr = [];
this.getAllTask();
}
getAllTask() {
this.crudService.getAllTask().subscribe(res => {
this.taskArr = res;
}, err => {
alert("Unable to get list of tasks");
});
}
addTask() {
this.taskObj.task_name = this.addTaskValue;
this.crudService.addTask(this.taskObj).subscribe(res => {
this.ngOnInit();
this.addTaskValue = '';
}, err => {
alert(err);
})
}
editTask() {
this.taskObj.task_name = this.editTaskValue;
this.crudService.editTask(this.taskObj).subscribe(res => {
this.ngOnInit();
}, err=> {
alert("Failed to update task");
})
}
deleteTask(etask : Task) {
this.crudService.deleteTask(etask).subscribe(res => {
this.ngOnInit();
}, err=> {
alert("Failed to delete task");
});
}
call(etask : Task) {
this.taskObj = etask;
this.editTaskValue = etask.task_name;
}
}
crud.service.ts
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http'
import { Task } from '../model/task';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class CrudService {
serviceUrl:string=""
constructor(private http : HttpClient) {
this.serviceUrl = "http://localhost:3000/tasks"
}
addTask(task : Task) : Observable<Task> {
return this.http.post<Task>(this.serviceUrl,task);
}
getAllTask() : Observable<Task[]> {
return this.http.get<Task[]>(this.serviceUrl);
}
deleteTask(task : Task) : Observable<Task> {
return this.http.delete<Task>(this.serviceUrl+'/'+task.id);
}
editTask(task : Task) : Observable<Task> {
return this.http.put<Task>(this.serviceUrl+'/'+task.id,task);
}
}
Find source code on git hub
Thanks, I have just been looking for information about this subject for a long time and yours is the best I’ve discovered till now. However, what in regards to the bottom line? Are you certain in regards to the supply?
The article provides a comprehensive overview of this topic. ❤️
Simply wish to say your article is as amazing. The clearness in your post is just nice and i could assume you’re an expert on this subject. Well with your permission let me to grab your feed to keep updated with forthcoming post. Thanks a million and please carry on the gratifying work.
Very interesting information!Perfect just what I was searching for!