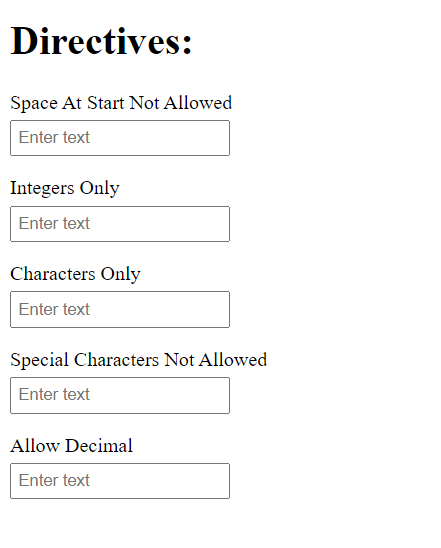
Steps to create directive
Step 1 create an angular application with command
ng new directiveApp
Step 2 create an directive with command
ng generate directive directiveName
Step 3 copy and paste the code given in above directive.ts file.
directive.ts
import { Directive,ElementRef,Input,HostListener } from '@angular/core';
@Directive({
selector: '[appDirectives]'
})
export class DirectivesDirective {
inputElement: ElementRef;
@Input('appDirectives')
appDirectives!: string;
arabicRegex = '[\u0600-\u06FF]';
constructor(el: ElementRef) {
this.inputElement = el;
}
@HostListener('keypress', ['$event']) onKeyPress(event: any) {
this.notAllowSpaceatFirst(event)
}
integerOnly(event: any) {
const e = <KeyboardEvent>event;
if (e.key === 'Tab' || e.key === 'TAB') {
return;
}
if (['1', '2', '3', '4', '5', '6', '7', '8', '9', '0'].indexOf(e.key) === -1) {
e.preventDefault();
}
}
noSpecialChars(event: any) {
const e = <KeyboardEvent>event;
if (e.key === 'Tab' || e.key === 'TAB') {
return;
}
let k;
k = event.keyCode; // k = event.charCode; (Both can be used)
if ((k > 64 && k < 91) || (k > 96 && k < 123) || k === 8 || k === 32 || (k >= 48 && k <= 57)) {
return;
}
const ch = String.fromCharCode(e.keyCode);
const regEx = new RegExp(this.arabicRegex);
if (regEx.test(ch)) {
return;
}
e.preventDefault();
}
onlyChars(event: any) {
const e = <KeyboardEvent>event;
if (e.key === 'Tab' || e.key === 'TAB') {
return;
}
let k;
k = event.keyCode; // k = event.charCode; (Both can be used)
if ((k > 64 && k < 91) || k === 8 || k === 32 || (k > 96 && k < 124)) {
return;
}
e.preventDefault();
}
allowDecimal(event: any) {
const e = <KeyboardEvent>event;
if (e.key === 'Tab' || e.key === 'TAB') {
return;
}
let k;
k = event.keyCode; // k = event.charCode; (Both can be used)
if ((k == 48) || (k == 49) || (k == 50) || (k == 51) ||
(k == 52) || (k == 53) || (k == 54) || (k == 55) ||
(k == 56) || (k == 57)) {
var arcontrol = new Array();
var temp = this.inputElement.nativeElement.value;
arcontrol = this.inputElement.nativeElement.value.split(".");
if (arcontrol.length == 1) {
if (arcontrol[0].length < 16) {
return;
}
else {
}
}
else {
return;
}
}
else if (k == 46) {
var sCount = new Array();
sCount = this.inputElement.nativeElement.value.split(".");
if ((sCount.length) - 1 == 1) {
}
else {
return;
}
}
e.preventDefault();
}
notAllowSpaceatFirst(event: any) {
if (event.target.selectionStart === 0 && event.code === "Space") {
event.preventDefault();
}
else {
if (this.appDirectives === 'integer') {
this.integerOnly(event);
} else if (this.appDirectives === 'noSpecialChars') {
this.noSpecialChars(event);
}
else if (this.appDirectives === 'onlyChars') {
this.onlyChars(event);
}
else if (this.appDirectives === 'allowDecimal') {
this.allowDecimal(event);
}
}
}
}
Component.ts
<h1>Directives:</h1>
<div>
<p>Space At Start Not Allowed</p>
<input appDirectives="" type="test" placeholder="Enter text">
</div>
<div>
<p>Integers Only</p>
<input appDirectives="integer" type="test" placeholder="Enter text">
</div>
<div>
<p>Characters Only</p>
<input appDirectives="onlyChars" type="test" placeholder="Enter text">
</div>
<div>
<p>Special Characters Not Allowed</p>
<input appDirectives="noSpecialChars" type="test" placeholder="Enter text">
</div>
<div>
<p>Allow Decimal</p>
<input appDirectives="allowDecimal" type="test" placeholder="Enter text">
</div>
Wow! This blog looks exactly like my old one! It’s on a entirely
different subject but it has pretty much the same layout
and design. Excellent choice of colors!
Best blog for developers compiler
I like this blog very much, Its a really nice office to
read and get info.